학습할 것
- 선택문
- if/else
- Switch/case
- 반복문
- for
- for-each
- while
- do-while
- 제어
- continue
- break
1. 선택문
if/else
- 참인지 거짓인지 확인하여 참일 경우에만 해당 블록 내부가 동작
(Ex. 홀수 짝수 분류)
1
2
3
4
5
6
7
8
9
10
11
12
|
public class Example {
public static void main(String[] args) {
int a = 4;
if((a & 1) == 0) {
System.out.println("짝수입니다.");
}
else {
System.out.println("홀수입니다.");
}
}
}
|
0~1은 변수 선언 및 저장이므로 2부터 if문 내부의 조건문 시작입니다.
여기서 볼 점은 if문에 대해 조건을 사용할 때와 달리 else를 사용하면 내부적으로 goto문을 사용한 다는 것을 볼 수 있습니다.
(Else if문을 포함한 예제)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
public class Example {
public static void main(String[] args) {
int a = 4;
if((a & 1) == 0) {
System.out.println("짝수입니다.");
}
else if((a & 1) == 1) {
System.out.println("홀수입니다.");
}
else {
System.out.println("???");
}
}
}
|
if문과 같이 else if문도 똑같이 동작하지만 else는 goto문이 동작하는 것을 볼 수 있습니다.
Switch/case
- 하나의 변수를 통해 선택을 하여 하나의 값을 지정하는 방식
- 위에서 차례로 순차적 접근
(예제 1. 성적)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
|
public class Example {
public static void main(String[] args) {
int score = 3;
switch(score) {
case 1:
case 2:
System.out.println("F");
case 3:
System.out.println("D");
break;
case 4: case 5:
System.out.println("C");
break;
case 7:
System.out.println("A");
break;
}
}
}
|
score의 값과 case에 매칭 하여 변경됩니다.
break가 없을 때에는 순차적으로 아래로 내려가서 자신의 값과 맞는 case문에서 실행합니다.
따라서 1~2이면 'F'가, 3이면 'D', 4~5이면 'C', 7이면 'A'가 나옵니다.
숫자로 했을 때에는 각 switch table이 생성되고 해당되는 case에 가서 실행이 되며 break문이 있으면 return(default) 부분으로 이동하며 마지막 case문에서는 default문이 없으면 바로 return으로 가는 것을 볼 수 있습니다.
(default문을 포함한 class)
default문이 없으면 default와 return이 같았던 부분과는 달리, default를 추가했을 때에는 break시 return으로 가며 default부분도 따로 생긴 것을 볼 수 있습니다.
(Ex. 성씨 출력)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
|
public class Example {
public static void main(String[] args) {
String first = "김";
switch(first) {
case "김":
case "이":
case "박":
System.out.println("김이박");
case "최":
System.out.println("최");
break;
case "한": case "서":
System.out.println("한서");
break;
case "정":
System.out.println("정");
break;
default:
System.out.println("???");
}
}
}
|
다음과 같이 loopup 테이블을 만들어서 각 String값을 매핑한 후
스위치 테이블을 통해 스위치문을 동작시키는 것을 볼 수 있습니다.
아래는 default문을 없애고 똑같이 실행을 해보았습니다.
사실 이 class파일을 본 이유는 좀 더 깊은 어셈블리단에서 보고 싶었지만 못하여 간단하게 컴파일러가 자동으로 최적화하는 것을 보고 싶었습니다. if문과 다르게 switch-case문은 간단한 숫자 범위부터 한글까지 테이블 형태로 만들어서 동작한 것을 알고 가면 좋을 거 같습니다.
추가적인 Switch 연산자의 경우 이전 포스트 3.연산자 마지막에서 더 볼 수 있습니다.
반복문
For
- 반복 횟수를 지정한 만큼 반복하는 문법
- for(초기화; 조건문; 증감자)
(예제, i가 5, j가 3 하나라도 만족할 때까지 동작)
1
2
3
4
5
6
7
|
public class Example {
public static void main(String[] args) {
for(int i=0, j=0; (i < 5) && (j <3); i++, j++) {
System.out.println("i:"+i+" j:"+j);
}
}
}
|

for-each
- 반복할 객체를 통해 각 요소를 출력 가능합니다.
- 배열 전체 요소를 한 번씩 접근합니다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
public class Example {
public static void main(String[] args) {
int[] arr = new int[5];
arr[0] = 0;
arr[1] = 2;
arr[2] = 4;
arr[3] = 6;
arr[4] = 8;
for(int e: arr) {
System.out.print(e+" ");
}
System.out.println();
for(var e: arr) {
System.out.print(e+" ");
}
}
}
|
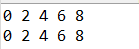
while
- 조건문을 통해서만 반복합니다.
- while(조건문)
- 조건문으로만 동작시키므로 무한루프에 빠질 위험이 있습니다.
1
2
3
4
5
6
7
8
9
|
public class Example {
public static void main(String[] args) {
int a = 0;
while(a<10) {
System.out.print(a++);
}
}
}
|
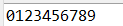
do-while
- 조건문을 통해서만 반복
- 처음 한 번은 while문을 실행합니다.
- while문이 구문 마지막에 있으며 조건을 통한 비교도 마지막에 합니다.
- do{}while(조건문);
1
2
3
4
5
6
7
8
|
public class Example {
public static void main(String[] args) {
int a = 0;
do {
System.out.print(a++);
}while(a<10);
}
}
|
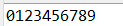
3. 제어
Continue
- 특정 조건이 만족하면 그 즉시 다음 반복으로 넘깁니다.
1
2
3
4
5
6
7
8
9
10
|
public class Example {
public static void main(String[] args) {
int a = 0;
for(int i=0; i <10; i++) {
System.out.println(i+":Continue 전");
if(i==5)continue;
System.out.println(i+":Continue 후");
}
}
}
|
Break
- 특정 조건이 만족하면 그 즉시 반복문을 벗어납니다.
1
2
3
4
5
6
7
8
9
10
|
public class Example {
public static void main(String[] args) {
int a = 0;
for(int i=0; i <10; i++) {
System.out.println(i+":Continue 전");
if(i==5)break;
System.out.println(i+":Continue 후");
}
}
}
|
'JAVA > 자바스터디' 카테고리의 다른 글
상속 (0) | 2022.11.13 |
---|---|
[자바스터디] 5. 클래스 (0) | 2022.01.06 |
[자바스터디] 3. 연산자 (0) | 2022.01.03 |
[자바스터디] 2. 변수 (0) | 2022.01.02 |
[자바스터디] 1.1 컴파일과 실행하는 법 (0) | 2021.12.27 |